Get started immediately and produce your first drawing within minutes. This crate is an excellent tool for learning and teaching the Rust programming language. Anyone of any age or skill level can learn how to create art with code!
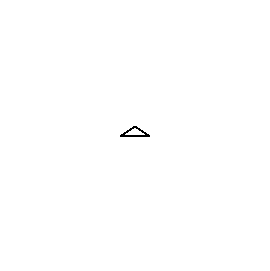
The Basic Concept
You control a turtle with a pen tied to its tail. As it moves across the screen, it draws the path that it follows. Change the turtle's speed, its pen's color, and more to create more complex drawings and animations.
This simple mental model is all you need to draw all kinds of pictures!
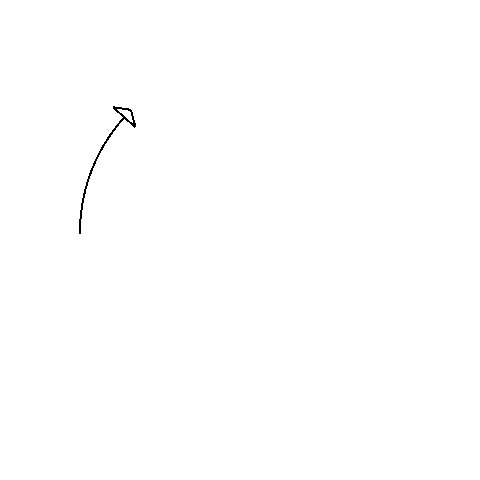
Easy to Learn Graphics
We designed Turtle to be incredibly easy to learn and user friendly. We leverage the power of Rust's advanced type system to make using Turtle as convenient for you as possible.
Use different colors, fills and other options to create anything you want! Turtle supports lots of different drawing commands so you can get really creative!
1
2
3
4
5
6
7
8
9
10
11
12
// This program draws a triangle
let mut turtle = Turtle::new();
turtle.set_pen_color("red");
turtle.set_fill_color("orange");
// Turn right 30 degrees
turtle.right(30.0);
for _ in 0..3 {
// Walk forward 150 steps
turtle.forward(150.0);
// Turn right 120 degrees
turtle.right(120.0);
}
Go From Basic To Advanced
Start from the most basic concepts, then quickly work your way up as you learn to draw more complex things.
1
2
3
4
5
6
7
8
9
10
// Draws what looks like several nested cubes
for i in 0..290 {
let i = i as f64;
turtle.set_pen_color(Color {
red: (i / 300.0 * 4.0) * 255.0 % 255.0,
green: 255.0, blue: 255.0, alpha: 1.0,
});
turtle.forward(i);
turtle.right(60.0);
}
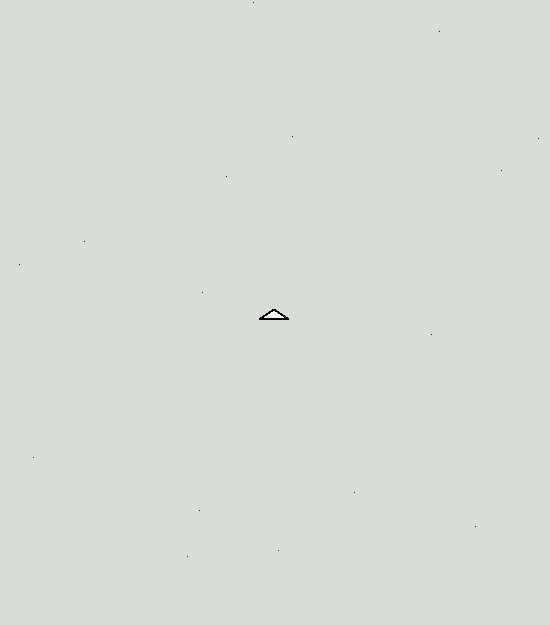